Part#
- class ansys.meshing.prime.Part(model, id, object_id, name)#
Part contains zonelets and topoentities.
Topoentities and zonelets are characterized by dimension of entities. Zonelets are a group of interconnected elements in a mesh. There are three types of zonelets. They are:
FaceZonelet: A group of interconnected face elements.
EdgeZonelet: A group of interconnected edge elements.
CellZonelet: A group of interconnected cell elements.
Topoentities represent connectivity information. Topoentities can be queried from higher order to lower order topoentities and vice versa. Topoentities have geometric representation which may be defined by splines or facets. The mesh generated on topoentities will be projected on geometry representation.
TopoFace: Topoentity representing surfaces.
TopoEdge: Topoentity representing curves.
TopoVolume: Topoentity representing volumes.
- Parameters:
Methods
Part.add_labels_on_topo_entities
(labels, ...)Add the given labels on the provided topoentities.
Part.add_labels_on_zonelets
(labels, zonelets)Add the given labels on the provided zonelets.
Part.add_topo_entities_to_zone
(zone_id, ...)Add topoentities to zone.
Part.add_volumes_to_zone
(zone_id, volumes)Add volumes to zone.
Part.add_zonelets_to_zone
(zone_id, zonelets)Add zonelets to zone.
Part.compute_closed_volumes
(params)Computes volume by identifying closed volumes defined by face zonelets of the part.
Part.compute_topo_volumes
(params)Compute topovolumes by identifying closed volumes defined by topofaces of the part.
Part.delete_topo_entities
(params)Delete topoentities of part controled by parameters.
Part.delete_volumes
(volumes, params)Delete volumes by deleting its face zonelets.
Part.delete_zonelets
(zonelets)Delete given face zonelets.
Part.extract_topo_volumes
(topo_faces, params)Extract topovolumes connected to given cap topofaces.
Part.extract_volumes
(face_zonelets, params)Extract volumes connected to given face zonelets.
Gets the adjacent facezonelets for the provided facezonelet ids.
Part.get_adjacent_volumes_of_volumes
(volumes)Get the adjacent volumes for the provided volume ids.
Get the cell zonelet ids in the part.
Get the edge zonelets of a part.
Get edge zonelet ids of labels with name matching the given name pattern.
Get all the edge zones of the part.
Part.get_face_zone_of_zonelet
(zonelet)Gets the face zone of given zonelet.
Get the face zonelets of a part.
Get face zonelet ids of labels with name matching the given name pattern.
Part.get_face_zonelets_of_volumes
(volumes)Get the face zonelets of given volumes.
Get ids of face zonelets of zones with name matching the given name pattern.
Get all the face zones of the part.
Get ids of face zones with name matching the given name pattern.
Get all labels on entities of part.
Part.get_labels_on_zonelet
(zonelet_id)Gets labels associated with zonelet.
Gets the name of the Part.
Gets the list of spline ids.
Part.get_summary
(params)Get the part summary.
Get the topoedges of a part.
Get topoedge ids of labels with name matching the given name pattern.
Get the topofaces of a part.
Get topoface ids of labels with name matching the given name pattern.
Get topoface ids of zones with name matching the given name pattern.
Get topovolumes of part.
Gets the unstructured surface spline for the part.
Part.get_volume_zone_of_volume
(volume)Gets the volume zone of given volume.
Get all the volume zones of the part.
Get ids of volume zones with name matching the given name pattern.
Get all the volumes of the part.
Part.get_volumes_of_face_zonelet
(face_zonelet)Get volume ids of given face zonelet.
Get volume ids of zones with name matching the given name pattern.
Part.merge_volumes
(volumes, params)Merge volumes by removing shared face zonelets.
Part.merge_zonelets
(zonelets, params)Merge zonelets.
Remove the given labels from the provided topoentities.
Part.remove_labels_from_zonelets
(labels, ...)Remove the given labels from the provided zonelets.
Part.remove_zone_on_topo_entities
(topo_entities)Removes zone on the given topoentities.
Part.remove_zone_on_volumes
(volumes)Removes zone on the given volumes.
Part.remove_zone_on_zonelets
(zonelets)Removes zone on the given zonelets.
Part.set_suggested_name
(name)Set the unique name for the part to a suggested name.
Attributes
Get the id of Part.
Get the name of Part.
Whether IDs of TopoEntities or zonelets are set to print along with the part summary.
Whether the mesh summary is set to print along with the part summary.
Examples using Part#
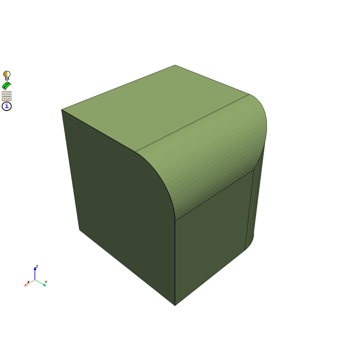
Convert data when importing and exporting mesh and CAD formats
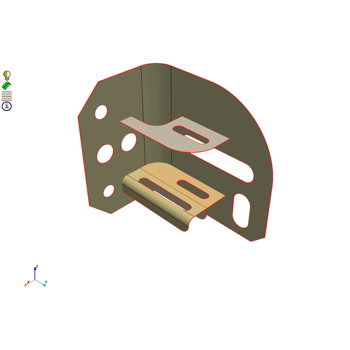
Mesh a mid-surfaced bracket for a structural analysis
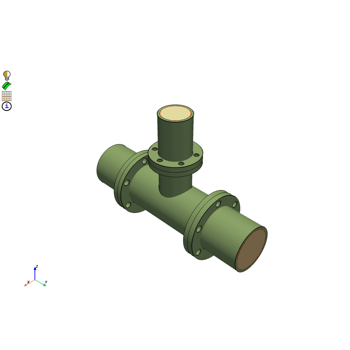
Mesh a pipe T-section for structural thermal and fluid flow analysis
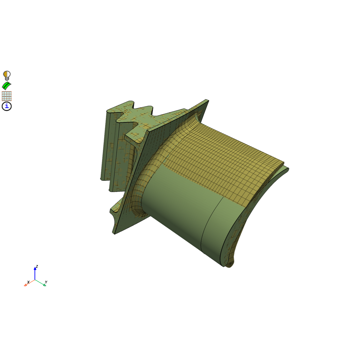
Morph a hexahedral mesh of a turbine blade to a new shape
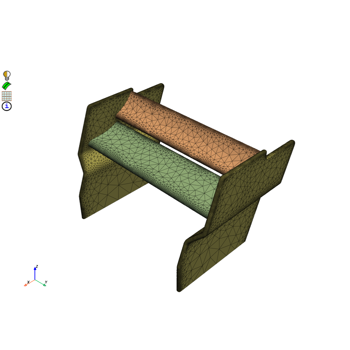
Mesh a generic F1 car rear wing for external aero simulation
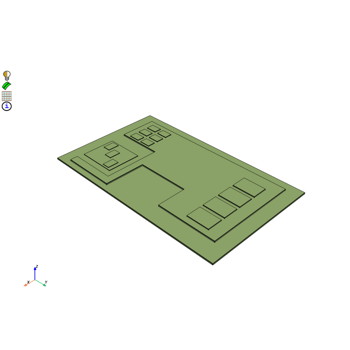
Mesh a generic PCB geometry with multiple hexa layers
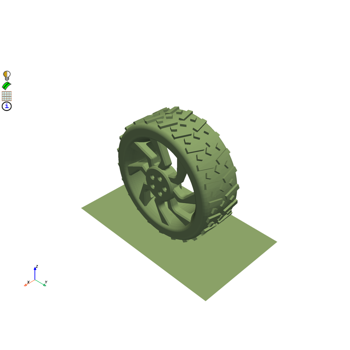
Create a contact patch for wrapping between a wheel and ground interface